Q: How can I see the bullet marks created with hit_hole on all the walls, regardless of their distance to the player?
A: The hole will appear on the wall only if the bullet is able to travel that much. Open weapons.wdl and set a bigger _bulletspeed for your weapon.
action weap_mg_animated
{
MY.__ROTATE = ON;
MY.__REPEAT = ON;
MY.__BOB = ON;
MY._OFFS_X = 45;
MY._OFFS_Y = 15;
MY._OFFS_Z = 15;
MY._AMMOTYPE = 1.99;
if (MY._WEAPONNUMBER == 0) { MY._WEAPONNUMBER = 2; }
MY._BULLETSPEED = 5000.01;
MY._FIRETIME = 2;
MY._FIREMODE = DAMAGE_SHOOT + HIT_HOLE + GUNFX_BRASS + GUNFX_ANIMATE + 0.20;
gun();
}
Q: How can I make that the crosshair visible at game start, without having to press the "K" key to show it?
A: Place this line at the end of your main function:
pan_cross_toggle();
Q: I was wondering if I could change a model's mesh depending on the video resolution that was set in the menu. This would increase the frame rate on weaker PCs.
A: Use something like this:
string high_poly = <highpoly.mdl>;
action my_model // attached to the low poly model
{
if (video_mode >= 8)
{
ent_morph (my, high_poly);
}
....................
}
Q: How can I create a flickering light?
A: Here's an example used for a fire:
action flickering_light
{
d3d_lightres = on;
while (1)
{
my.lightrange = 70 + random(30);
my.lightred = 150 + random(100);
my.lightgreen = 150;
my.lightblue = 50;
waitt (2);
}
}
Q: How can I speed up or slow down player's walking speed?
A: Open movement.wdl, find these lines and change the values:
var strength[3] = 5,4,75; // default ahead, side, jump strength
var astrength[3] = 7,5,2; // default pan, tilt, roll strength
Q: I would like to change the bitmap for a panel at runtime. Is it possible?
A: Use this piece of code:
bmap first_pcx = <first.pcx>;
bmap second_pcx = <second.pcx>;
panel my_pan
{
bmap = first_pcx;
pos_x = 0;
pos_y = 0;
flags = refresh, d3d, visible;
}
function change_bitmap()
{
my_pan.bmap = second_pcx;
}
on_q change_bitmap;
Q: How can I get unlimited ammo for one of my weapons?
A: Place these lines at the end of your main function:
while (1)
{
if (ammo1 < 50) {ammo1 = 50;}
wait (1);
}
and you will get unlimited ammo for every weapon that uses ammo1 (weapon_mg_animated, etc).
Q: How do I make the player invulnerable for 20 seconds?
A: Paste the code below at the end of your office.wdl fle:
function invulnerability()
{
player.event = null;
sleep (20);
player.event = fight_event;
}
on_i = invulnerability; // I = invulnerability
Q: How do I assign a name to a player in multiplayer mode?
A: Run the game using "-pl your_name":
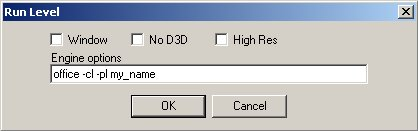
You can see the name of the client using a simple text definition:
text player_txt // displays the name of the player in multiplayer mode
{
font = _a4font;
pos_x = 0;
pos_y = 100;
string = player_name;
flags = visible;
}
|